Quick Start - Area of Interest (AOI) Query¶
In this section, we will find and request a pre-defined area of interest (AOI) and use it to initiate a Environmental Intelligence (EI): Geospatial APIs raster query.
In order to run this notebook, ibmpairs
, geopandas
, geoplot
, numpy
and matplotlib
must be installed to your python environment using your package manager of choice, e.g. with pip:
pip install ibmpairs geopandas geoplot numpy matplotlib
Search for AOI¶
An AOI, in Geospatial APIs, is a pre-defined labelled spatial definition that can be specified as the spatial extent (area) of a raster query; usually a jurisdiction (e.g. country, state etc).
To use an AOI in a raster query, we must find an area that we wish to query; to do so, we can use the SDK method query.search_aois
:
[1]:
import os
import ibmpairs.query as query
import ibmpairs.client as client
import geopandas
import geoplot
import PIL.Image
import numpy as np
import matplotlib.pyplot as plt
# It is best practice not to include secrets in source code so
# we read an api key, tenant id and org id from operating system
# environment variables.
EI_API_KEY = os.environ.get('EI_API_KEY')
EI_TENANT_ID = os.environ.get('EI_TENANT_ID')
EI_ORG_ID = os.environ.get('EI_ORG_ID')
# Authenticate and get a client object.
ei_client = client.get_client(api_key = EI_API_KEY,
tenant_id = EI_TENANT_ID,
org_id = EI_ORG_ID)
# Search for AOIs that contain the word 'texas'
aois_list = query.search_aois('texas')
aois_list
2024-06-19 10:03:54 - paw - INFO - The client authentication method is assumed to be OAuth2.
2024-06-19 10:03:54 - paw - INFO - Legacy Environment is False
2024-06-19 10:03:54 - paw - INFO - The authentication api key type is assumed to be IBM Cloud IAM, because the api key prefix 'PHX' is not present.
2024-06-19 10:03:55 - paw - INFO - Authentication success.
2024-06-19 10:03:55 - paw - INFO - HOST: https://api.ibm.com/geospatial/run/na/core/v3
[1]:
id | key | name | |
---|---|---|---|
0 | 51575 | missouri.texas | Missouri.Texas |
1 | 52132 | oklahoma.texas | Oklahoma.Texas |
2 | 52653 | texas.anderson | Texas.Anderson |
3 | 52558 | texas.andrews | Texas.Andrews |
4 | 52661 | texas.angelina | Texas.Angelina |
... | ... | ... | ... |
509 | 52553 | texas.yoakum | Texas.Yoakum |
510 | 52595 | texas.young | Texas.Young |
511 | 52769 | texas.zapata | Texas.Zapata |
512 | 52758 | texas.zavala | Texas.Zavala |
513 | 164 | usa-texas | USA - Texas |
514 rows × 3 columns
The search includes the results that contain the word texas
; the usa-texas
key represents the AOI of the state of Texas (USA), the texas.*
entries represent the counties of the state of Texas.
To get the metadata information about the usa-texas
area of interest (id: 164) it can be requested with query.get_aoi
.
[2]:
texas_id = int(aois_list.loc[aois_list['key'] == 'usa-texas', 'id'].iloc[0])
texas = query.get_aoi(texas_id)
texas
2024-06-19 10:03:56 - paw - INFO - The AOI metadata for 164 was retreived.
[2]:
{
"bbox": [
25.795808134,
-106.683192366,
36.543596825,
-93.457684889
],
"geojson": "{\"type\":\"Polygon\",\"coordinates\":[[[-106.683192366,31.942887307],[-106.623555771,32.051088309],[-103.106759183,32.052422446],[-103.069255984,36.518767545],[-100.001664892,36.543596825],[-99.947481351,34.629539979],[-99.666860972,34.42584217],[-99.443502852,34.421964197],[-99.35225105,34.498731218],[-99.168595192,34.365424006],[-99.151419194,34.258301545],[-98.617954477,34.207820825],[-98.45306249,34.106891538],[-98.364816544,34.190188117],[-98.09945857,34.196541332],[-98.046652334,34.041269506],[-97.952756011,34.041201779],[-97.864138897,33.907847955],[-97.676628909,34.038434165],[-97.405805152,33.873028749],[-97.212875245,33.955774758],[-97.038044097,33.898091427],[-96.926673431,34.011772333],[-96.735380541,33.884608678],[-96.680475368,33.961655112],[-96.564887797,33.942126555],[-96.337457867,33.777559085],[-95.955516065,33.938561937],[-95.839855327,33.89476448],[-95.606920256,33.994652663],[-95.381475661,33.918893627],[-95.231846792,34.014910591],[-94.698494179,33.743140481],[-94.004066235,33.594411362],[-93.985199649,32.032647195],[-93.791098661,31.826988238],[-93.767547467,31.588362385],[-93.662551777,31.545530928],[-93.457684889,31.033670436],[-93.700113749,30.381833203],[-93.665049423,30.045410481],[-93.890130492,29.821797319],[-93.799781583,29.639425381],[-94.056090317,29.624317626],[-94.657152118,29.389436631],[-94.718038976,29.280154556],[-95.159355468,29.06646069],[-95.213790288,28.942762424],[-95.50016826,28.761183681],[-96.186041713,28.443188984],[-96.289079434,28.601154175],[-96.432821374,28.529113479],[-96.34373839,28.450730681],[-96.359955447,28.313841319],[-97.006743803,27.814142144],[-97.337113518,27.096559989],[-97.124072365,25.942387258],[-97.439608822,25.795808134],[-97.679904807,25.978401576],[-98.21225533,26.007020435],[-98.836320548,26.322054341],[-99.140934462,26.383114572],[-99.327120372,26.827148364],[-99.50162411,27.00994253],[-99.592395872,27.573469602],[-99.909497886,27.761719649],[-99.981046649,27.94715027],[-100.336999739,28.249004006],[-100.838189521,29.20917135],[-101.100663006,29.430928588],[-101.292388839,29.487132103],[-101.454690557,29.711166129],[-102.071783739,29.735317214],[-102.315415773,29.823623154],[-102.367950031,29.721216833],[-102.654036124,29.685139842],[-102.839343066,29.326617625],[-102.827541717,29.198036795],[-103.128945492,28.935570357],[-103.298967082,28.939999442],[-104.072183054,29.285652478],[-104.567370224,29.640927351],[-104.936146093,30.541708409],[-105.420535727,30.813004765],[-106.026575894,31.351101793],[-106.244681586,31.439332696],[-106.415717092,31.691927148],[-106.653129721,31.785506674],[-106.683192366,31.942887307]],[[-97.239626055,26.128786906],[-97.407263982,26.696945754],[-97.446677021,26.958965578],[-97.515484394,26.938223626],[-97.410651734,26.466128023],[-97.239626055,26.128786906]],[[-97.450153219,27.076390578],[-97.450269297,27.080311662],[-97.450868042,27.078870744],[-97.450153219,27.076390578]],[[-97.291085769,27.754783882],[-97.21678344,27.726390349],[-97.187452766,27.763276336],[-97.343308575,27.793643851],[-97.291085769,27.754783882]]]}",
"id": 164,
"key": "usa-texas",
"name": "USA - Texas"
}
In order to check the AOI has the desired shape, it can be loaded into a geopandas dataframe and plotted with geoplot:
[3]:
texas_geopandas = geopandas.read_file(texas.geojson, driver='GeoJSON')
gx = geoplot.polyplot(texas_geopandas.geometry)
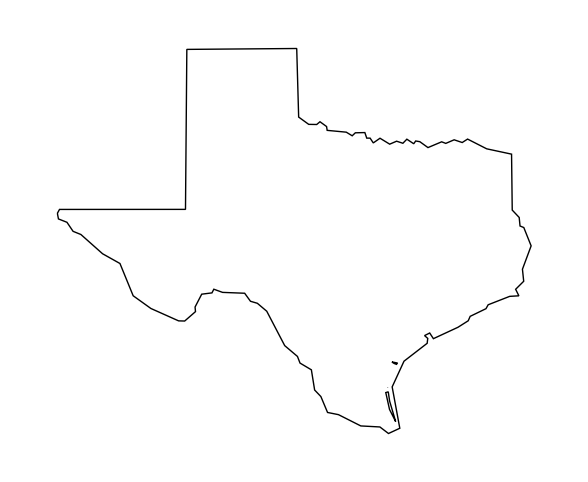
Query with AOI¶
Once it has been verified that the AOI is of the desired location for the query, we can run a raster query (see Quick Start- Raster Query) with the texas AOI id as the specified spatial definition:
[4]:
# ERA 5 Temperature query for Texas
ERA5_texas_query_json = {
"layers": [
{
"id": "49423",
"type": "raster"
}
],
"name": "ERA5 1h Query for Texas for 2024-04-01",
"spatial": {
"aoi": texas_id,
"type": "poly"
},
"temporal": {
"intervals": [
{
"end": "2024-04-01T01:00:00Z",
"start": "2024-04-01T00:00:00Z"
}
]
}
}
ERA5_texas_query_result = query.submit_check_status_and_download(ERA5_texas_query_json)
2024-06-19 10:03:56 - paw - INFO - TASK: submit_check_status_and_download STARTING.
2024-06-19 10:03:57 - paw - INFO - The query was successfully submitted with the id: 1718755200_32637744.
2024-06-19 10:03:58 - paw - INFO - The query 1718755200_32637744 has the status Queued.
2024-06-19 10:04:28 - paw - INFO - The query 1718755200_32637744 has the status Succeeded.
2024-06-19 10:04:28 - paw - INFO - The query 1718755200_32637744 was successful after checking the status.
2024-06-19 10:04:59 - paw - INFO - The query 1718755200_32637744 has the status Succeeded.
2024-06-19 10:04:59 - paw - INFO - The query 1718755200_32637744 was successful after checking the status.
2024-06-19 10:04:59 - paw - INFO - The query download folder is set to the path /Users/ibmpairs/tutorials/notebooks/quickstart/download/.
2024-06-19 10:05:01 - paw - INFO - The query 1718755200_32637744 is a zip.
2024-06-19 10:05:01 - paw - INFO - The query file for 1718755200_32637744 will be downloaded to the following path /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.zip.
2024-06-19 10:05:01 - paw - INFO - The query file for 1718755200_32637744 was successfully downloaded to /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.zip.
2024-06-19 10:05:01 - paw - INFO - The query zip /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.zip will be unzipped to the following path /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.
2024-06-19 10:05:01 - paw - INFO - The query zip /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.zip was successfully unzipped to /Users/ibmpairs/tutorials/notebooks/quickstart/download/1718755200_32637744.
2024-06-19 10:05:31 - paw - INFO - TASK: submit_check_status_and_download COMPLETED.
[5]:
# Find layer files to load from downloaded zip.
files = ERA5_texas_query_result.list_files()
[6]:
# Display the resulting image
array = np.array(PIL.Image.open(files[1]))
plt.figure(figsize = (20, 12))
plt.imshow(array, cmap = 'hot_r', vmin = 293, vmax = 307)
plt.title('Temperature (Kelvin)')
plt.colorbar()
plt.show()
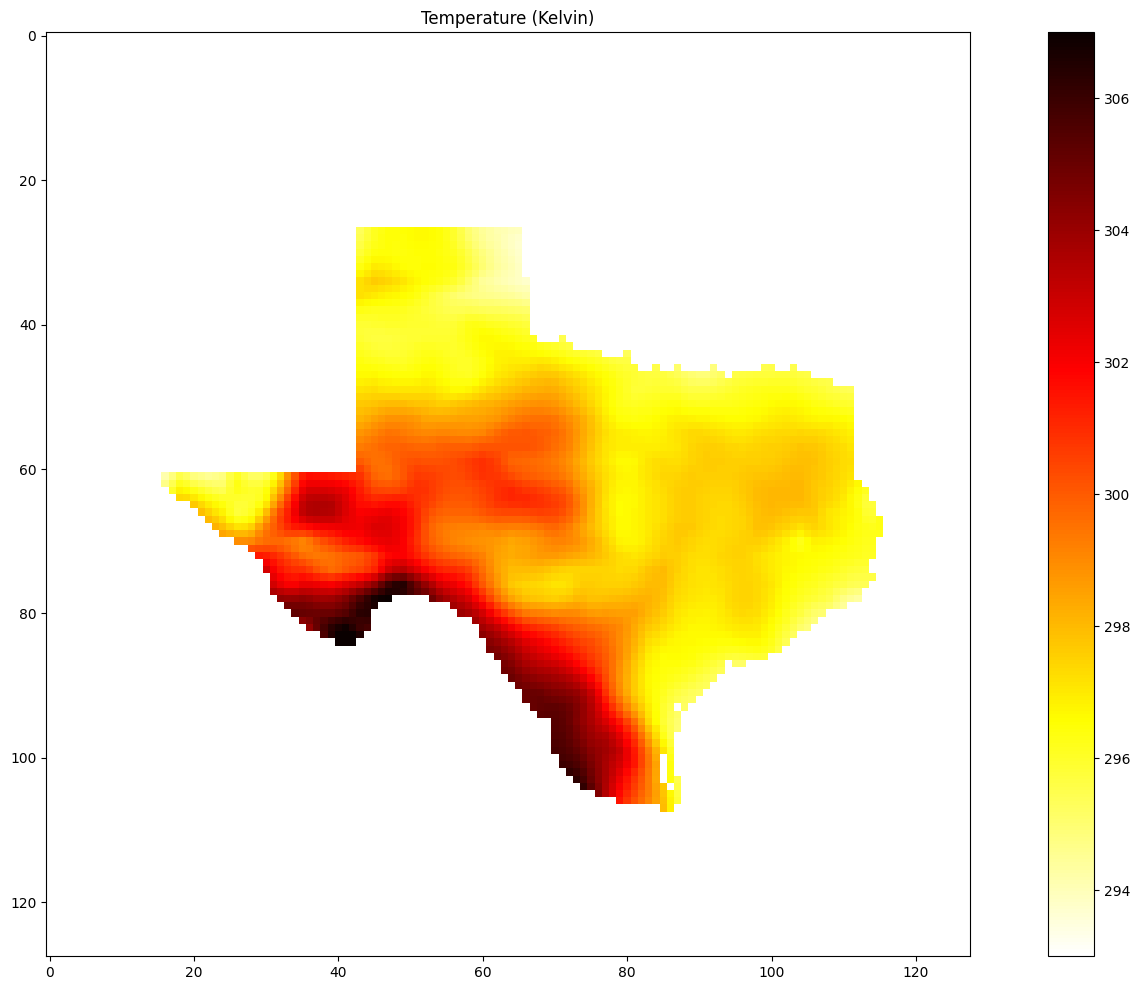