An abstract main class representing an underlying HE library & scheme, configured, initialized, and ready to start working. More...
#include <HeContext.h>
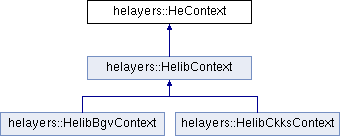
Public Member Functions | |
HeContext () | |
Constructs an empty object. | |
HeContext (const HeContext &src)=delete | |
Copy constructor. More... | |
HeContext & | operator= (const HeContext &src)=delete |
Copy from another object. More... | |
virtual void | init (const HeConfigRequirement &req)=0 |
Internal use. | |
virtual bool | isConfigRequirementFeasible (const HeConfigRequirement &req) const =0 |
Internal use. | |
virtual std::shared_ptr< AbstractCiphertext > | createAbstractCipher ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractPlaintext > | createAbstractPlain ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractEncoder > | getEncoder ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractFunctionEvaluator > | getFunctionEvaluator () |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractBitwiseEvaluator > | getBitwiseEvaluator () |
Do not use. Should be made private. | |
const HeTraits & | getTraits () |
Returns an HeTraits object containing various properties of the underlying scheme. | |
virtual bool | hasSecretKey () const =0 |
Returns whether this context contains a secret key. More... | |
virtual int | slotCount () const =0 |
The number of slots in each CTile (ciphertext) or PTile (plaintext) created over this context. | |
virtual int | getTopChainIndex () const =0 |
Returns the highest available chain index (for schemes where it is applicable). | |
virtual int | getSecurityLevel () const =0 |
Returns the security level supplied by this context. | |
virtual void | printSignature (std::ostream &out=std::cout) const =0 |
Prints a summary of library details and configuration params. More... | |
virtual void | debugPrint (const std::string &title="", int verbose=0, std::ostream &out=std::cout) const =0 |
Prints detailed information for debug purposes. More... | |
virtual std::shared_ptr< std::vector< uint64_t > > | getModulusChain () const |
Returns the modulus chain bit length for each prime in the chain (where applicable). | |
virtual void | save (std::ostream &out, bool withSecretKey) |
Saves this context to a stream in binary form. More... | |
virtual void | load (std::istream &in) |
Loads context saved by the save() method. More... | |
virtual void | saveSecretKey (std::ostream &out)=0 |
Save secret key to the given ostream. More... | |
virtual void | loadSecretKey (std::istream &in)=0 |
Load secret key from the given istream. More... | |
void | saveToFile (const std::string &fileName, bool withSecretKey) |
Saves this context to a file in binary form. More... | |
void | loadFromFile (const std::string &fileName) |
Loads context saved by the saveToFile() method. More... | |
void | saveSecretKeyToFile (const std::string &fileName) |
save secret key to the given file. More... | |
void | loadSecretKeyFromFile (const std::string &fileName) |
load secret key from the given file. More... | |
virtual double | getDefaultScale () const |
Returns default scale used in encoding (where applicable). | |
virtual void | setDefaultScale (double v) |
Sets the default scale to be used in encoding (where applicable). More... | |
virtual std::string | getLibraryName () const =0 |
Returns the name of the underlying library. | |
virtual std::string | getSchemeName () const =0 |
Returns the name of the underlying scheme. | |
virtual std::string | getSignature () const |
Returns a signature of the context that distinguishes it enough to be able to load previously stored contexts based on their signatures. | |
virtual std::shared_ptr< JsonWrapper > | getEstimatedLatencies () const |
For internal use. | |
virtual std::shared_ptr< HeContext > | clone () const |
Returns an uninitialized context of the same type. More... | |
Static Public Member Functions | |
static std::shared_ptr< HeContext > | loadHeContextFromFile (const std::string &fileName) |
Returns a pointer to a context initialized from file. More... | |
static std::shared_ptr< HeContext > | loadHeContext (std::istream &in) |
Returns a pointer to a context initialized from stream. More... | |
static bool | internalRegisterContext (const HeContext *context) |
Registers a context object for the purpose of dynamic loading. More... | |
Protected Attributes | |
HeTraits | traits |
Detailed Description
An abstract main class representing an underlying HE library & scheme, configured, initialized, and ready to start working.
Upon initaliation, use on of the inheriting concrete classes such as HelibCkksContext. Then continue with a generic reference to HeContext to allow scheme obliviousness
This class bundles together all the data structures required for performing HE operations, including the keys. It either contains a public/private key pair, allowing all operations including decryption, or just the public key allowing everything but decryption.
It is used as input in constructor of many other classes in this library. For example, to create a ciphertext using the library represent by an HeContext, write: CTile c(he);
It can be further used to query various properties of the underlying scheme and library.
Constructor & Destructor Documentation
◆ HeContext()
|
delete |
Copy constructor.
- Parameters
-
[in] src Object to copy.
Member Function Documentation
◆ clone()
|
virtual |
Returns an uninitialized context of the same type.
Used for dynamic type loading among others.
Reimplemented in helayers::HelibCkksContext.
◆ debugPrint()
|
pure virtual |
Prints detailed information for debug purposes.
- Parameters
-
[in] title Title to print along with the information. [in] verbose Verbosity level [in] out Output stream to print to
Implemented in helayers::HelibContext.
◆ hasSecretKey()
|
pure virtual |
Returns whether this context contains a secret key.
If not, decryption and other operations relying on decryption will not be available (will throw an exception).
Implemented in helayers::HelibContext.
◆ internalRegisterContext()
|
static |
Registers a context object for the purpose of dynamic loading.
Don't call this directly. Use REGISTER_CONTEXT (see above)
◆ load()
|
virtual |
Loads context saved by the save() method.
- Parameters
-
[in] out input stream to read from
Reimplemented in helayers::HelibCkksContext, helayers::HelibContext, and helayers::HelibBgvContext.
◆ loadFromFile()
void helayers::HeContext::loadFromFile | ( | const std::string & | fileName | ) |
Loads context saved by the saveToFile() method.
- Parameters
-
[in] fileName file to read from
◆ loadHeContext()
|
static |
Returns a pointer to a context initialized from stream.
Context type is dynamically determined by content of stream.
- Parameters
-
[in] in stream to read from
◆ loadHeContextFromFile()
|
static |
Returns a pointer to a context initialized from file.
Context type is dynamically determined by content of file.
- Parameters
-
[in] fileName file to read from
◆ loadSecretKey()
|
pure virtual |
Load secret key from the given istream.
- Parameters
-
[in] istream the binary stream to load from.
- Exceptions
-
runtime_error if this HeContext already has a secret key. i.e. hasSecretKey() is ture.
Implemented in helayers::HelibContext.
◆ loadSecretKeyFromFile()
void helayers::HeContext::loadSecretKeyFromFile | ( | const std::string & | fileName | ) |
load secret key from the given file.
- Parameters
-
[in] fileName the path of the file to load from
- Exceptions
-
runtime_error if this HeContext already has a secret key. i.e. hasSecretKey() is ture.
◆ operator=()
Copy from another object.
- Parameters
-
[in] src Object to copy.
◆ printSignature()
|
pure virtual |
Prints a summary of library details and configuration params.
- Parameters
-
[in] out output stream to write to
Implemented in helayers::HelibContext, and helayers::HelibBgvContext.
◆ save()
|
virtual |
Saves this context to a stream in binary form.
- Parameters
-
[in] out output stream to write to [in] withSecretKey whether to include the secret key
Reimplemented in helayers::HelibContext.
◆ saveSecretKey()
|
pure virtual |
Save secret key to the given ostream.
- Parameters
-
[out] ostream the binary stream to save to.
- Exceptions
-
runtime_error if this HeContext doesn't have a secret key. i.e. hasSecretKey() is false.
Implemented in helayers::HelibContext.
◆ saveSecretKeyToFile()
void helayers::HeContext::saveSecretKeyToFile | ( | const std::string & | fileName | ) |
save secret key to the given file.
- Parameters
-
[out] fileName the path of the file to save to.
- Exceptions
-
runtime_error if this HeContext doesn't have a secret key. i.e. hasSecretKey() is false.
◆ saveToFile()
void helayers::HeContext::saveToFile | ( | const std::string & | fileName, |
bool | withSecretKey | ||
) |
Saves this context to a file in binary form.
- Parameters
-
[in] fileName file to write to [in] withSecretKey whether to include the secret key
◆ setDefaultScale()
|
inlinevirtual |
Sets the default scale to be used in encoding (where applicable).
- Parameters
-
[in] v the default scale
The documentation for this class was generated from the following files:
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/HeContext.h
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/HeContext.cpp