helayers::HelibBgvContext Class Reference
An implementation of HeContext for BGV scheme in HElib. More...
#include <HelibBgvContext.h>
Inheritance diagram for helayers::HelibBgvContext:
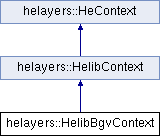
Public Member Functions | |
void | init (unsigned long p, unsigned long m, unsigned long r, unsigned long L) |
void | init (const HelibConfig &conf) override |
Initializes context with given configuration. More... | |
void | init (const HelibConfig &conf, helib::Context *userContext, helib::SecKey *userSecretKey, helib::PubKey *userPublicKey) |
Initializes context with given context and keys. More... | |
virtual std::shared_ptr< AbstractCiphertext > | createAbstractCipher () |
Returns a pointer to HelibBgvCiphertext, initialized with this HelibBgvContext. | |
virtual std::shared_ptr< AbstractPlaintext > | createAbstractPlain () |
Returns a pointer to HelibBgvPlaintext, initialized with this HelibBgvContext. | |
std::shared_ptr< AbstractFunctionEvaluator > | getFunctionEvaluator () override |
Returns a pointer to HelibBgvNativeFunctionEvaluator, initialized with this HelibBgvContext. | |
std::shared_ptr< AbstractEncoder > | getEncoder () override |
Returns a pointer to HelibBgvEncoder, initialized with this HelibBgvContext. | |
void | printSignature (std::ostream &out=std::cout) const override |
Prints a summary of library details and configuration params. More... | |
virtual const helib::EncryptedArray & | getEncryptedArray () |
Returns the EncryptedArray used to perform operation on ciphertexts and plaintexts that are initialized with this scheme. | |
void | load (std::istream &in) |
Loads this HelibBgvcontext from the given istream. More... | |
std::string | getSchemeName () const override |
Returns the name of this scheme (i.e. "BGV"). | |
![]() | |
void | init (const HeConfigRequirement &req) override |
Internal use. | |
void | initPreset (HelibPreset preset) |
Initalizes with a given preset. More... | |
int | getTopChainIndex () const override |
Returns the highest available chain index (for schemes where it is applicable). | |
int | slotCount () const override |
The number of slots in each CTile (ciphertext) or PTile (plaintext) created over this context. | |
int | getSecurityLevel () const override |
Returns the security level supplied by this context. | |
bool | hasSecretKey () const override |
Returns whether this context contains a secret key. More... | |
const helib::PubKey & | getPublicKey () const |
const helib::SecKey & | getSecretKey () const |
const helib::Context & | getContext () const |
bool | getMirrored () const |
void | setMirrored (bool v) |
void | printSignature (std::ostream &out=std::cout) const override |
Prints a summary of library details and configuration params. More... | |
void | debugPrint (const std::string &title="", int verbose=0, std::ostream &out=std::cout) const override |
Prints detailed information for debug purposes. More... | |
void | save (std::ostream &out, bool withSecretKey) override |
Saves this context to a stream in binary form. More... | |
void | load (std::istream &in) override |
Loads context saved by the save() method. More... | |
void | saveSecretKey (std::ostream &out) override |
Save secret key to the given ostream. More... | |
void | loadSecretKey (std::istream &in) override |
Load secret key from the given istream. More... | |
std::string | getLibraryName () const override |
Returns the name of the underlying library. | |
bool | isConfigRequirementFeasible (const HeConfigRequirement &req) const override |
Internal use. | |
![]() | |
HeContext () | |
Constructs an empty object. | |
HeContext (const HeContext &src)=delete | |
Copy constructor. More... | |
HeContext & | operator= (const HeContext &src)=delete |
Copy from another object. More... | |
virtual std::shared_ptr< AbstractBitwiseEvaluator > | getBitwiseEvaluator () |
Do not use. Should be made private. | |
const HeTraits & | getTraits () |
Returns an HeTraits object containing various properties of the underlying scheme. | |
virtual std::shared_ptr< std::vector< uint64_t > > | getModulusChain () const |
Returns the modulus chain bit length for each prime in the chain (where applicable). | |
void | saveToFile (const std::string &fileName, bool withSecretKey) |
Saves this context to a file in binary form. More... | |
void | loadFromFile (const std::string &fileName) |
Loads context saved by the saveToFile() method. More... | |
void | saveSecretKeyToFile (const std::string &fileName) |
save secret key to the given file. More... | |
void | loadSecretKeyFromFile (const std::string &fileName) |
load secret key from the given file. More... | |
virtual double | getDefaultScale () const |
Returns default scale used in encoding (where applicable). | |
virtual void | setDefaultScale (double v) |
Sets the default scale to be used in encoding (where applicable). More... | |
virtual std::string | getSignature () const |
Returns a signature of the context that distinguishes it enough to be able to load previously stored contexts based on their signatures. | |
virtual std::shared_ptr< JsonWrapper > | getEstimatedLatencies () const |
For internal use. | |
virtual std::shared_ptr< HeContext > | clone () const |
Returns an uninitialized context of the same type. More... | |
Additional Inherited Members | |
![]() | |
static std::shared_ptr< HelibContext > | create (HelibPreset preset) |
Creates a new HelibContext for either CKKS or BGV, based on a preset configuration. More... | |
![]() | |
static std::shared_ptr< HeContext > | loadHeContextFromFile (const std::string &fileName) |
Returns a pointer to a context initialized from file. More... | |
static std::shared_ptr< HeContext > | loadHeContext (std::istream &in) |
Returns a pointer to a context initialized from stream. More... | |
static bool | internalRegisterContext (const HeContext *context) |
Registers a context object for the purpose of dynamic loading. More... | |
![]() | |
HelibConfig | config |
helib::Context * | context = NULL |
helib::SecKey * | secretKey = NULL |
helib::PubKey * | publicKey = NULL |
long | nslots = 0 |
bool | mirrored = false |
![]() | |
HeTraits | traits |
Detailed Description
An implementation of HeContext for BGV scheme in HElib.
It can be either initialized via parameters, via an HelibConfig, or loaded from a file.
It is recommended not to use directly after initialization, but use an HeContext reference instead.
It is currently in beta version, as not all BGV operators are covered.
Member Function Documentation
◆ init() [1/2]
|
overridevirtual |
Initializes context with given configuration.
- Parameters
-
[in] conf user configuration
Reimplemented from helayers::HelibContext.
◆ init() [2/2]
void helayers::HelibBgvContext::init | ( | const HelibConfig & | conf, |
helib::Context * | userContext, | ||
helib::SecKey * | userSecretKey, | ||
helib::PubKey * | userPublicKey | ||
) |
Initializes context with given context and keys.
- Parameters
-
[in] conf user configuration [in] userContext user context [in] userSecretKey user secret key [in] userPublicKey user public key
◆ load()
|
virtual |
Loads this HelibBgvcontext from the given istream.
- Parameters
-
[in] istream The istream to load from.
Reimplemented from helayers::HeContext.
◆ printSignature()
|
overridevirtual |
Prints a summary of library details and configuration params.
- Parameters
-
[in] out output stream to write to
Implements helayers::HeContext.
The documentation for this class was generated from the following files:
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/helib/HelibBgvContext.h
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/helib/HelibBgvContext.cpp