An implementation of HeContext API with concrete subclasses for HElib scheme. More...
#include <HelibContext.h>
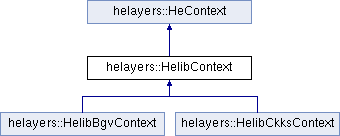
Public Member Functions | |
void | init (const HeConfigRequirement &req) override |
Internal use. | |
void | initPreset (HelibPreset preset) |
Initalizes with a given preset. More... | |
virtual void | init (const HelibConfig &conf) |
Initialize context with given configuration. More... | |
int | getTopChainIndex () const override |
Returns the highest available chain index (for schemes where it is applicable). | |
int | slotCount () const override |
The number of slots in each CTile (ciphertext) or PTile (plaintext) created over this context. | |
int | getSecurityLevel () const override |
Returns the security level supplied by this context. | |
bool | hasSecretKey () const override |
Returns whether this context contains a secret key. More... | |
const helib::PubKey & | getPublicKey () const |
const helib::SecKey & | getSecretKey () const |
const helib::Context & | getContext () const |
bool | getMirrored () const |
void | setMirrored (bool v) |
void | printSignature (std::ostream &out=std::cout) const override |
Prints a summary of library details and configuration params. More... | |
void | debugPrint (const std::string &title="", int verbose=0, std::ostream &out=std::cout) const override |
Prints detailed information for debug purposes. More... | |
void | save (std::ostream &out, bool withSecretKey) override |
Saves this context to a stream in binary form. More... | |
void | load (std::istream &in) override |
Loads context saved by the save() method. More... | |
void | saveSecretKey (std::ostream &out) override |
Save secret key to the given ostream. More... | |
void | loadSecretKey (std::istream &in) override |
Load secret key from the given istream. More... | |
std::string | getLibraryName () const override |
Returns the name of the underlying library. | |
bool | isConfigRequirementFeasible (const HeConfigRequirement &req) const override |
Internal use. | |
![]() | |
HeContext () | |
Constructs an empty object. | |
HeContext (const HeContext &src)=delete | |
Copy constructor. More... | |
HeContext & | operator= (const HeContext &src)=delete |
Copy from another object. More... | |
virtual std::shared_ptr< AbstractCiphertext > | createAbstractCipher ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractPlaintext > | createAbstractPlain ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractEncoder > | getEncoder ()=0 |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractFunctionEvaluator > | getFunctionEvaluator () |
Do not use. Should be made private. | |
virtual std::shared_ptr< AbstractBitwiseEvaluator > | getBitwiseEvaluator () |
Do not use. Should be made private. | |
const HeTraits & | getTraits () |
Returns an HeTraits object containing various properties of the underlying scheme. | |
virtual std::shared_ptr< std::vector< uint64_t > > | getModulusChain () const |
Returns the modulus chain bit length for each prime in the chain (where applicable). | |
void | saveToFile (const std::string &fileName, bool withSecretKey) |
Saves this context to a file in binary form. More... | |
void | loadFromFile (const std::string &fileName) |
Loads context saved by the saveToFile() method. More... | |
void | saveSecretKeyToFile (const std::string &fileName) |
save secret key to the given file. More... | |
void | loadSecretKeyFromFile (const std::string &fileName) |
load secret key from the given file. More... | |
virtual double | getDefaultScale () const |
Returns default scale used in encoding (where applicable). | |
virtual void | setDefaultScale (double v) |
Sets the default scale to be used in encoding (where applicable). More... | |
virtual std::string | getSchemeName () const =0 |
Returns the name of the underlying scheme. | |
virtual std::string | getSignature () const |
Returns a signature of the context that distinguishes it enough to be able to load previously stored contexts based on their signatures. | |
virtual std::shared_ptr< JsonWrapper > | getEstimatedLatencies () const |
For internal use. | |
virtual std::shared_ptr< HeContext > | clone () const |
Returns an uninitialized context of the same type. More... | |
Static Public Member Functions | |
static std::shared_ptr< HelibContext > | create (HelibPreset preset) |
Creates a new HelibContext for either CKKS or BGV, based on a preset configuration. More... | |
![]() | |
static std::shared_ptr< HeContext > | loadHeContextFromFile (const std::string &fileName) |
Returns a pointer to a context initialized from file. More... | |
static std::shared_ptr< HeContext > | loadHeContext (std::istream &in) |
Returns a pointer to a context initialized from stream. More... | |
static bool | internalRegisterContext (const HeContext *context) |
Registers a context object for the purpose of dynamic loading. More... | |
Protected Attributes | |
HelibConfig | config |
helib::Context * | context = NULL |
helib::SecKey * | secretKey = NULL |
helib::PubKey * | publicKey = NULL |
long | nslots = 0 |
bool | mirrored = false |
![]() | |
HeTraits | traits |
Detailed Description
An implementation of HeContext API with concrete subclasses for HElib scheme.
Member Function Documentation
◆ create()
|
static |
Creates a new HelibContext for either CKKS or BGV, based on a preset configuration.
See list of presets in HelibConfig.h
- Parameters
-
preset Preset configuration
◆ debugPrint()
|
overridevirtual |
Prints detailed information for debug purposes.
- Parameters
-
[in] title Title to print along with the information. [in] verbose Verbosity level [in] out Output stream to print to
Implements helayers::HeContext.
◆ hasSecretKey()
|
inlineoverridevirtual |
Returns whether this context contains a secret key.
If not, decryption and other operations relying on decryption will not be available (will throw an exception).
Implements helayers::HeContext.
◆ init()
|
virtual |
Initialize context with given configuration.
- Parameters
-
conf Configuration details
Reimplemented in helayers::HelibCkksContext, and helayers::HelibBgvContext.
◆ initPreset()
void helayers::HelibContext::initPreset | ( | HelibPreset | preset | ) |
Initalizes with a given preset.
See list of presets in HelibConfig.h
- Parameters
-
preset Preset configuration name
◆ load()
|
overridevirtual |
Loads context saved by the save() method.
- Parameters
-
[in] out input stream to read from
Reimplemented from helayers::HeContext.
◆ loadSecretKey()
|
overridevirtual |
Load secret key from the given istream.
- Parameters
-
[in] istream the binary stream to load from.
- Exceptions
-
runtime_error if this HeContext already has a secret key. i.e. hasSecretKey() is ture.
Implements helayers::HeContext.
◆ printSignature()
|
overridevirtual |
Prints a summary of library details and configuration params.
- Parameters
-
[in] out output stream to write to
Implements helayers::HeContext.
◆ save()
|
overridevirtual |
Saves this context to a stream in binary form.
- Parameters
-
[in] out output stream to write to [in] withSecretKey whether to include the secret key
Reimplemented from helayers::HeContext.
◆ saveSecretKey()
|
overridevirtual |
Save secret key to the given ostream.
- Parameters
-
[out] ostream the binary stream to save to.
- Exceptions
-
runtime_error if this HeContext doesn't have a secret key. i.e. hasSecretKey() is false.
Implements helayers::HeContext.
The documentation for this class was generated from the following files:
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/helib/HelibContext.h
- /opt/IBM/FHE-distro/ML-HElib/src/helayers/hebase/helib/HelibContext.cpp